글에 들어가며
Spring Boot 3.0.2 Project에서 Jayspt 3.0.5 를 활용하여서 Configuration 파일(application.properties 혹은 application.yaml) 의 문자열을 암호화하는 방식에 대해서 담아보겠습니다.
아래의 사이트는 Jayspt 의 Reference 사이트입니다.
https://github.com/ulisesbocchio/jasypt-spring-boot#use-you-own-custom-encryptor
GitHub - ulisesbocchio/jasypt-spring-boot: Jasypt integration for Spring boot
Jasypt integration for Spring boot. Contribute to ulisesbocchio/jasypt-spring-boot development by creating an account on GitHub.
github.com
Prequisite
- Spring Boot 3.0.2
구현과정
1. jayspt 3.0.5 버전을 다운받습니다.
implementation 'com.github.ulisesbocchio:jasypt-spring-boot-starter:3.0.5' //For application yaml Encryption
아래의 글 덕분에 jayspt 를 3.0.5 로 버전업시키면서 오류를 해결했습니다.
spring boot 3.0 이상에서는 jayspt를 3.0.5 버전을 사용해야합니다.
https://velog.io/@gmtmoney2357/spring-boot-3%EC%97%90%EC%84%9C-jasypt-%EC%97%90%EB%9F%AC
spring boot 3에서 jasypt 에러
jasypt을 사용하기 위해 구글 서칭을 하면, 많은 블로그와 사이트에서 예제 코드를 확인할 수 있다.대부분의 코드들이 아래와 같이 3.0.4를 사용하는 것을 확인할 수 있다.하지만 스프링 부트 3에서
velog.io
2. 암호화하고자하는 파일을 application.yml에 등록합니다.
https://www.devglan.com/online-tools/jasypt-online-encryption-decryption
Programming Blog Article Feeds as per your Interest | DevGlan
Best programming article feeds as per your Interest on different technologies. Subscribe to any technology and explore the best articles from around the web.
www.devglan.com
helloworld를 secretKey를 사용하여 암호화시켰습니다.
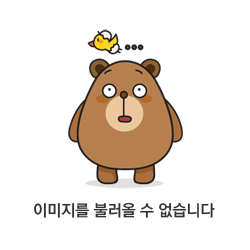
이때, 아래 3번과정에서 나올 이야기이지만, jasypt는 2가지의 알고리즘 방식을 제공하는데 아래의 알고리즘을 사용할것이기에 위의 사이트에서 접속해서 암호화를 시킵니다.
config.setAlgorithm("PBEWithMD5AndDES");
2. jasypt를 사용하기 위해 @Configuration으로 등록하고 @Bean설정을 하기 위해 config 파일을 추가합니다. 저는 다음의 경로와 같이 추가했습니다. [\src\main\java\com\seminarhub\config\JasyptConfig.java ]
@Configuration @EnableEncryptableProperties //@EncryptablePropertySources(@EncryptablePropertySource("classpath:/application.yml")) public class JasyptConfig { @Value("${jasypt.encryptor.password}") private String jasyptEncryptorPassword; /** * https://github.com/ulisesbocchio/jasypt-spring-boot#use-you-own-custom-encryptor * https://www.devglan.com/online-tools/jasypt-online-encryption-decryption * Pass Password And Set it as a JVM System Property Environment Example : java -Djasypt.encryptor.password=password -jar target/jasypt-spring-boot-demo-0.0.1-SNAPSHOT.jar * Pass Password by Particular Jasypt Library Environment Example : java --jasypt.encryptor.password=password -jar target/jasypt-spring-boot-demo-0.0.1-SNAPSHOT.jar */ @Bean("jasyptStringEncryptor") public StringEncryptor stringEncryptor() { System.out.println("HELLO JASYPT:"+jasyptEncryptorPassword); PooledPBEStringEncryptor encryptor = new PooledPBEStringEncryptor(); SimpleStringPBEConfig config = new SimpleStringPBEConfig(); config.setPassword(jasyptEncryptorPassword); // config.setAlgorithm("PBEWITHHMACSHA512ANDAES_256"); config.setAlgorithm("PBEWithMD5AndDES"); config.setKeyObtentionIterations("1000"); config.setPoolSize("1"); config.setProviderName("SunJCE"); config.setSaltGeneratorClassName("org.jasypt.salt.RandomSaltGenerator"); config.setIvGeneratorClassName("org.jasypt.iv.RandomIvGenerator"); config.setStringOutputType("base64"); encryptor.setConfig(config); return encryptor; } }
- jasyptEncryptorPassword는 아래의 그림과 같이 property가 아닌 환경변수로 넣어서 실행시킵니다.

3. 암호화하고자하는 값을 삽입합니다.
spring: secret: key: ENC(암호화한 값을 삽입합니다.)
4. 테스트해보기
@SpringBootTest public class JasyptTest { @DisplayName("Jasypt Encryption Test") @Test public void testEncryptionAndDecryption() { // given // when StandardPBEStringEncryptor encryptor = new StandardPBEStringEncryptor(); encryptor.setPassword("mySecretPassword"); String originalValue = "myData"; String encryptedValue = encryptor.encrypt(originalValue); System.out.println("Encrypted Value: " + encryptedValue); String decryptedValue = encryptor.decrypt(encryptedValue); System.out.println("Decrypted Value: " + decryptedValue); // then assert decryptedValue.equals(originalValue); } }
- 위의 코드를 통해서 정상적으로 Jasypt가 동작하는지 확인할 수 있습니다.
추가 정보
암호화할려는 문장에 '@' 가 포함되어있으면 Spring 에서 해당 언어 부분이 깨져서 올바르게 Deocde되지 않았었습니다.
해당사항 확인하시어 주시기바랍니다.
참고
Jasypt 를 적용할 때 주의할 점
https://www.devglan.com/online-tools/jasypt-online-encryption-decryption해당 사이트를 통해서 암호화를 했을 경우에는 암호화 알고리즘을 PBEWithMD5AndDES 로 설정해야 한다. Jasypt 설정으
velog.io